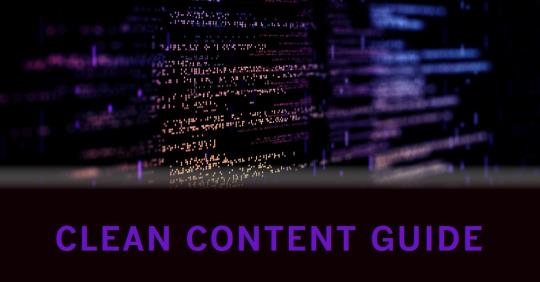
Clean Content: A Guide to Removing HTML Tags for Better Readability
Created on 29 July, 2024 • 295 views • 4 minutes read
Learn how to remove HTML tags for better readability and data analysis. This guide covers methods using regex, Beautiful Soup, JavaScript, and best practices for clean, tag-free content.
Clean Content: A Guide to Removing HTML Tags for Better Readability
In the digital age, content is king. But what happens when your valuable content is buried beneath a sea of HTML tags? Whether you're a content creator, web developer, or data analyst, knowing how to strip HTML tags from your text is an essential skill. This guide will walk you through the process of cleaning your content, ensuring better readability and improved user experience.
Why Remove HTML Tags?
Before we dive into the how-to, let's understand why removing HTML tags is crucial:
- Improved Readability: Plain text is easier to read and process, especially when working with large amounts of data.
- Data Cleaning: For data analysis or migration, you often need clean, tag-free text.
- Content Repurposing: When moving content from web to print or other formats, HTML tags become unnecessary.
- SEO Optimization: Clean content can be more effectively analyzed by search engines.
- Accessibility: Screen readers and other assistive technologies work better with clean text.
Methods to Remove HTML Tags
1. Using Regular Expressions (Regex)
Regular expressions are powerful tools for pattern matching and text manipulation. Here's a simple regex pattern to remove HTML tags:
python
Copy
import re
def remove_html_tags(text):
clean = re.compile('<.*?>')
return re.sub(clean, '', text)
# Example usage
html_text = "<p>This is <b>bold</b> text.</p>"
clean_text = remove_html_tags(html_text)
print(clean_text) # Output: This is bold text.
2. Using Beautiful Soup (Python)
Beautiful Soup is a popular Python library for parsing HTML and XML documents:
python
Copy
from bs4 import BeautifulSoup
def remove_tags_bs4(html):
soup = BeautifulSoup(html, "html.parser")
return soup.get_text()
# Example usage
html_text = "<p>This is <b>bold</b> text.</p>"
clean_text = remove_tags_bs4(html_text)
print(clean_text) # Output: This is bold text.
3. Using JavaScript
For client-side tag removal, you can use JavaScript:
javascript
Copy
function removeTags(html) {
let tmp = document.createElement("DIV");
tmp.innerHTML = html;
return tmp.textContent || tmp.innerText || "";
}
// Example usage
let htmlText = "<p>This is <b>bold</b> text.</p>";
let cleanText = removeTags(htmlText);
console.log(cleanText); // Output: This is bold text.
4. Online Tools
For quick, one-off cleaning tasks, online HTML strippers can be handy. However, be cautious with sensitive data. Some reputable options include:
- HTML Cleaner (https://html-cleaner.com/)
- HTML Strip (https://www.textfixer.com/html/html-strip-tags.php)
Best Practices for HTML Tag Removal
- Preserve Important Formatting: Sometimes, you may want to keep certain formatting. Consider replacing tags like <b> or <i> with markdown or other lightweight markup.
- Handle Special Characters: Pay attention to HTML entities (like &, <, etc.) and decode them appropriately.
- Maintain Structure: If the structure is important, consider replacing some tags with line breaks or other separators.
- Clean Whitespace: After removing tags, you might end up with excess whitespace. Use additional regex or string methods to clean this up.
- Test Thoroughly: Always test your tag removal process with various types of HTML content to ensure it works as expected.
Advanced Considerations
Dealing with Scripts and Styles
When removing HTML tags, be extra cautious with <script> and <style> tags. These often contain content that you don't want in your final text:
python
Copy
import re
def remove_html_and_scripts(text):
# First, remove script and style elements
text = re.sub(r'<(script|style).*?</\1>(?s)', '', text)
# Then, remove remaining HTML tags
text = re.sub(r'<.*?>', '', text)
return text
Handling Nested Tags
Sometimes, simple regex patterns might struggle with deeply nested tags. In such cases, using an HTML parser like Beautiful Soup is often more reliable.
The Impact on SEO
Removing HTML tags can significantly impact your SEO efforts:
- Content Analysis: Clean text allows search engines to better understand your content.
- Keyword Density: Without tags, your true keyword density becomes apparent.
- Snippet Generation: Clean text can lead to more accurate and appealing search snippets.
However, remember that HTML tags also provide important context to search engines. The goal is to clean the content for analysis or repurposing, not necessarily for the live web version of your content.
Conclusion
Removing HTML tags is a crucial skill in the toolkit of any web professional. Whether you're cleaning up content for analysis, preparing text for a new format, or just trying to improve readability, the methods outlined in this guide will help you achieve clean, tag-free content.
Remember, the choice of method depends on your specific needs, the volume of content you're dealing with, and the environment you're working in. Always test your chosen method thoroughly and be mindful of preserving the essential meaning and structure of your content.
Popular posts
-
Learning Morse Code: Techniques and Tools for Beginners• 1,112 views
-
-
-
smoke time calculator• 941 views
-